This is a short set up guide to get you going with the ST7789 display using a standard Arduino (ATMega328p). If you want to use this display with an ESP32, then there is a separate guide here.
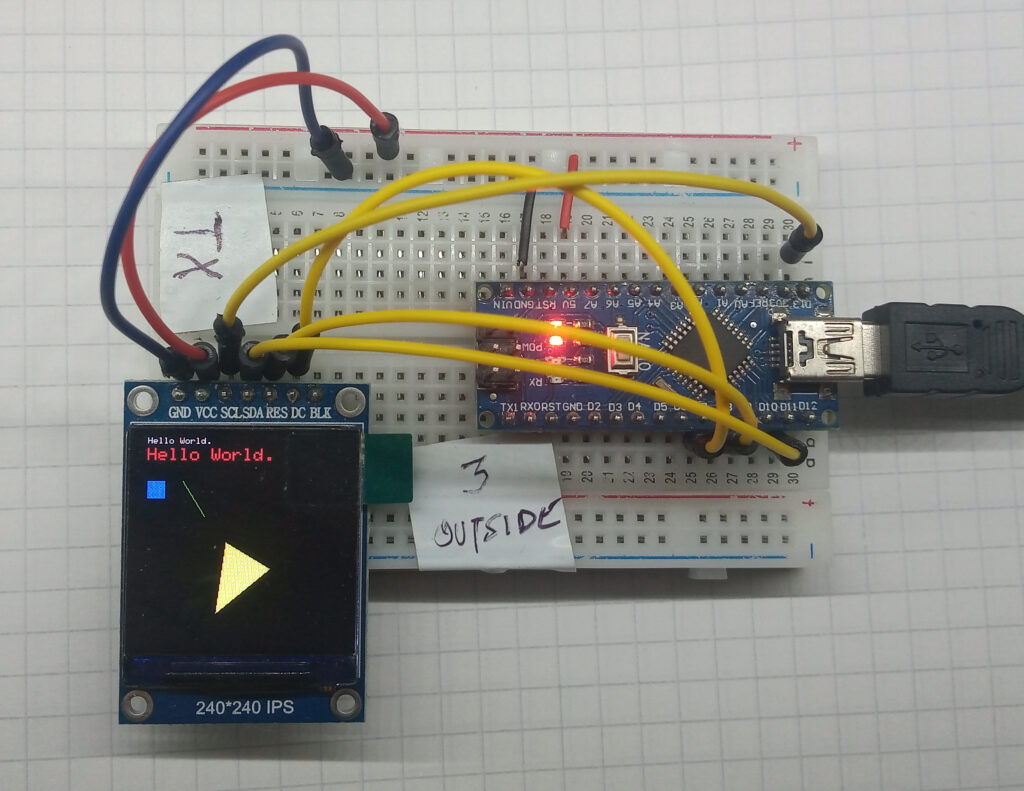
This display was used for the Weather Station project as well as creating an Arduino Racing game.
Hardware
This display is a 240×240 pixel, 16-bit (65k) colour TFT display with a ST7789 driver.
The “LiDAR night vision” project goes in to more detail about the colour.
The active LCD panel measures 23.4 x 23.4mm giving a pixel pitch of 0.0975mm, giving about 105pix/mm2. Decent.
The hook up is straight forward:
- BLK (not connected)
- DC > Arduino D7
- RES > Arduino D8
- SDA > Arduino D11 (SPI MOSI)
- SCL > Arduino D13 (SPI CLK)
- VCC > 5V (3.3V will not work reliably)
- GND > Arduino GND
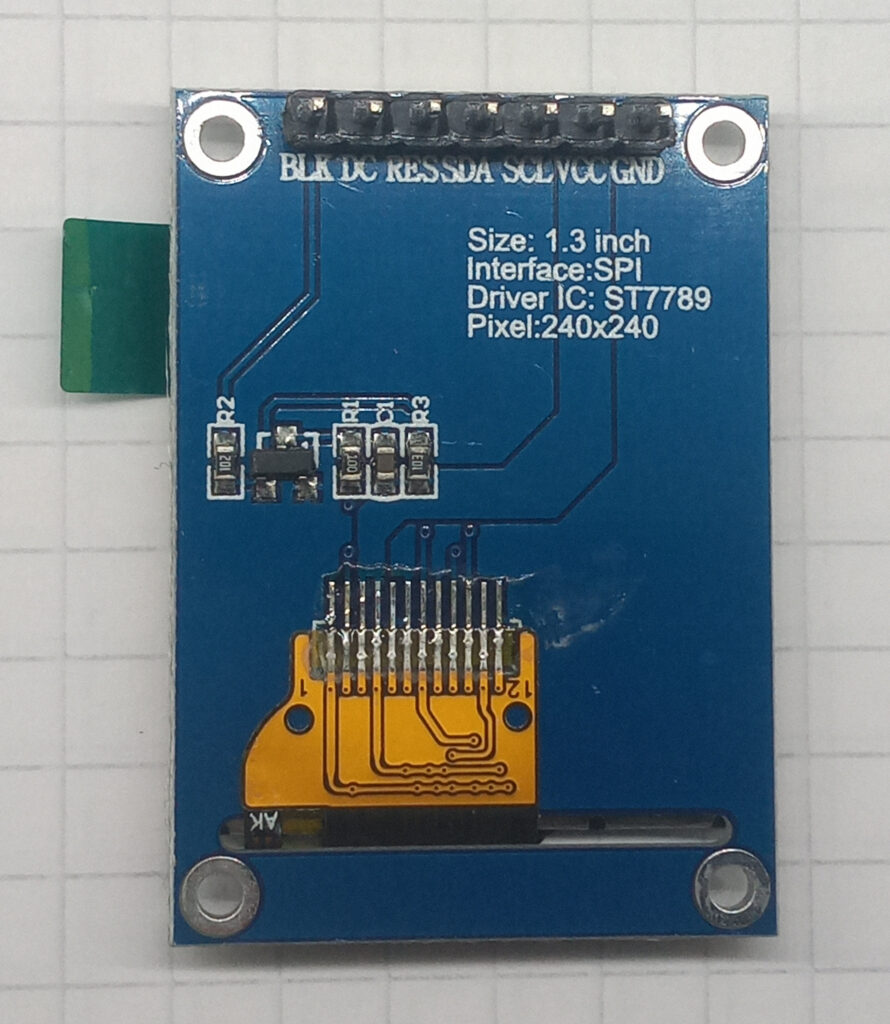
Software
As always, someone has done the donkey work for us and there are a select few libraries for this display. This example uses the ST7789_Fast library by cbm80amiga. As you guessed, it’s faster than the standard Adafruit ST7735/ST7789 library.
Both of the above libraries are built off the ever faithful Adafruit_GFX library, this means the standard drawLine(), fillRect(), etc, functions work.
Below is a basic section of code to demonstrate how to setup the display and carry out some simple tasks.
#include <SPI.h>
#include <Adafruit_GFX.h>
#include <Arduino_ST7789_Fast.h>
#define SCR_WD 240 // OLED display width, in pixels
#define SCR_HT 240 // OLED display height, in pixels
#define TFT_DC 7
#define TFT_RST 8
Arduino_ST7789 tft = Arduino_ST7789(TFT_DC, TFT_RST);
void setup() {
tft.begin();
tft.fillScreen(BLACK); //equivalent to clear screen
tft.setTextColor(WHITE);
}
void loop() {
tft.setCursor(10, 0);
tft.setTextSize(1);
tft.print("Hello World.");
tft.setCursor(10, 12);
tft.setTextSize(2);
tft.setTextColor(RED);
tft.print("Hello World.");
tft.drawLine(50, 50, 75, 90, GREEN);
tft.fillRect(10, 50, 20, 20, BLUE);
tft.fillTriangle(100 , 120, 150, 150, 90, 200, YELLOW);
while (1);
}
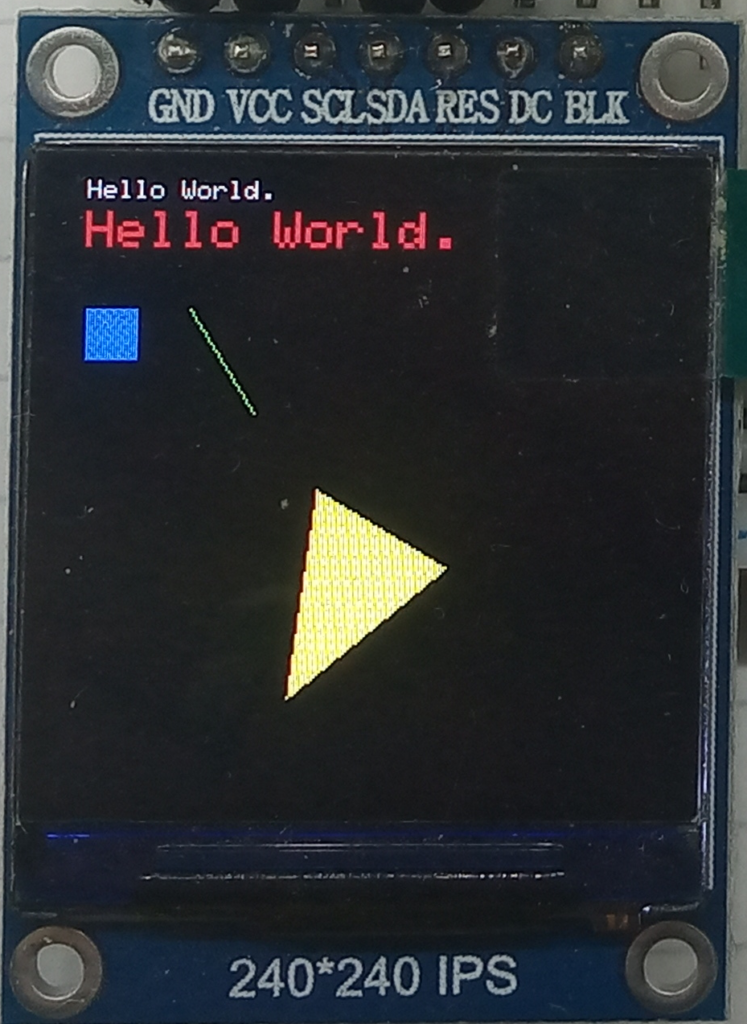
The colour palate is defined in the library’s .h header file. The defaults are as follows:
#define BLACK 0x0000
#define BLUE 0x001F
#define RED 0xF800
#define GREEN 0x07E0
#define CYAN 0x07FF
#define MAGENTA 0xF81F
#define YELLOW 0xFFE0
#define WHITE 0xFFFF
There is also the ability to convert RGB:
#define GREY RGBto565(128,128,128)
#define LGREY RGBto565(160,160,160)
#define DGREY RGBto565( 80, 80, 80)
#define LBLUE RGBto565(100,100,255)
#define DBLUE RGBto565( 0, 0,128)
Fin.
Page created: 04/06/2024